Recently, together with the B-I-T GmbH Information and processes from Hanover worked on implementing the electronic data exchange with the statutory health insurance funds and the pension insurance from one application.
Here, a combination of authenticated data transmission of both signed and encrypted messages is used. PKI technologies are used in all these cases.
The message format used is here documented.
Schematic flow of communication
The plaintext message is an XML-like structure (named eXTra), which is not described in detail here. This article is purely about the necessary PKI operations.
See also article "Cryptography basics„.
Signed message
The plain text message present in the eXTra format is stored in a Cryptographic Message Syntax (CMS, also known as PKCS#7) SignedData data structure embedded. This message is signed with the sender's private key to ensure the integrity and non-repudiation of the message.
Encrypted signed message
The signed message is then stored in a CMS (PKCS#7) EnvelopedData data structure and encrypted with the public keys of the recipients (one or more). Thus, the confidentiality of the message is now also guaranteed.
Schematically, the message now looks something like this:
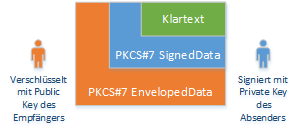
Data transmission of the encrypted signed message
The encrypted signed message is now sent or retrieved via one of the following ways:
- Via a web service in the case of pension insurance.
- By e-mail in the case of statutory health insurance.
In the following, only pension insurance will be considered, but in the case of statutory health insurance, the processes are largely the same.
The pension insurance company uses the following web services:
Environment | Address |
---|---|
Productive environment | https://itsg.eservice-drv.de/SPoC/ExtraService |
Test environment | https://itsg.eservicet-drv.de/SPoC/ExtraService |
Schematically, the following operations must be performed throughout the entire communication chain:
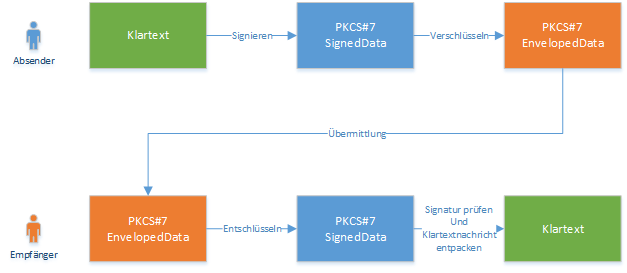
Implementation
Our first approach was to write a command line application in C# that does the creation/verification of the signatures and the encryption/decryption of the messages. This would have the advantage of being able to access the Windows certificate API and thus secure the certificates used on a smartcard or trusted platform module in the backend, for example.
C# (and PowerShell) are unfortunately ruled out, because the required CmsSigner class, the padding scheme cannot be specified, and the SHI RSASSA-PSS (PKCS#1 Version 2.1) prescribes.
The tools of choice are therefore OpenSSL and cURL. Here, too, there is the option of using a smartcard in the backend via the PKCS#11 interface, for example. For the sake of simplicity, however, the procedure with simple key files is described below.
Conversion to the appropriate file formats
First, the certificates must be in the appropriate format.
The following assumptions are made:
- The certificate used for signing and decrypting is in BASE64-encoded DER format (PEM).
- The certificate's private key is also in BASE64-encoded DER format (PEM) without a password.
- A file is available containing all the certification authority certificates involved, also in BASE64-encoded DER format (PEM).
Sign message
The following command is used to change the data stored in the file out_msg_plain.txt and the resulting signed message is saved in the file out_msg_signed.bin stored. To sign the message, the certificate of the sender (sender.pem) is used, to which the private key (sender.key) must be present.
openssl ^ cms ^ -sign ^ -binary ^ -in out_msg_plain.txt ^ -text ^ -nodetach -outform DER ^ -signer sender.pem ^ -certfile CAChain.pem ^ -inkey sender.key ^ -out out_msg_signed.bin ^ -keyopt rsa_padding_mode:pss ^ -nosmimecap
To improve the readability of the command, line breaks of the Windows command line have been entered.
The arguments used have the following meaning:
Argument | Description |
---|---|
cms | OpenSSL shall generate a CMS data structure (PKCS#7). |
-sign | This is a SignedData data structure. |
-binary | The read-in data is to be processed in binary format. |
-in out_msg_plain.txt | The specified message is to be read in. |
-text | The output message is accompanied by headers for the MIME message. |
-nodetach | The signed data should not be encoded in multipart/signed MIME type. |
-outform THE | The generated CMS data structure is to be output DER-coded (binary). |
-signer sender.pem | The generated CMS data structure is to be signed with the identity contained in the specified certificate. |
-certfile CAChain.pem | Contains the certificate chain of the used certificates to be able to establish the trust status to them. |
-inkey sender.key | Reference to the private key of the sender certificate. |
-out out_msg_signed.bin | The generated CMS data structure is to be output to the specified file. |
-keyopt rsa_padding_mode:pss | The padding mode to be used is the RSASSA-PSS mode required by SHI. |
-nosmimecap | By default, OpenSSL appends a S/MIME Capabilities Extension to the data structure (since a signed mail is identically structured), this behavior is prevented with this option. |
Encrypt message
At this point, it must be mentioned that in live operation, we are of course both the sender and the recipient of messages. In this case, we use the same certificate and private key for sending and receiving messages. In the following, however, the file name "empfaenger.pem" is used differently, since we want to simulate sending and receiving the same message in a development or test environment, for example.
The following command is used to change the data stored in the file out_msg_signed.bin encrypts the stored signed message and writes the resulting signed message to the file out_msg_encrypted.bin stored. For encryption, the certificate of the recipient (receiver.pem) without a private key is required.
openssl ^ cms ^ -encrypt ^ -binary ^ -in out_msg_signed.bin ^ -aes-256-cbc ^ -out msg_encrypted.bin ^ -outform DER ^ -recip empfaenger.pem ^ -keyopt rsa_padding_mode:oaep ^ -keyopt rsa_oaep_md:sha256
To improve the readability of the command, line breaks of the Windows command line have been entered.
The arguments used have the following meaning:
Argument | Description |
---|---|
cms | OpenSSL shall generate a CMS data structure (PKCS#7). |
-encrypt | It is an encrypted EnvelopedData data structure. |
-binary | The read-in data is to be processed in binary format. |
-in out_msg_signed.bin | The specified message is to be read in. |
-aes-256-cbc | The AES algorithm with 256-bit key length is to be used for encryption. |
-out msg_encrypted.bin | The generated CMS data structure is to be output to the specified file. |
-outform THE | The generated CMS data structure is to be output DER-coded (binary). |
-recip recipient.pem | Specifies the certificate of the recipient of the message. The message is encrypted with the corresponding public key. The parameter can be specified several times if the message is to be sent to multiple recipients. |
-keyopt rsa_padding_mode:oaep | The SHI interface description requires that the OAEP-AES padding mode be used. |
-keyopt rsa_oaep_md:sha256 | The SHI interface description requires that the SHA-256 hash algorithm be used. |
Please note: If the sender does not add himself to the list of recipients, he will not be able to decrypt the generated message. Usually there is no reason for this, but this fact can easily lead to confusion.
Decrypt message
The following command is used to change the data stored in the file in_msg_encrypted.bin decrypts the stored message and writes the signed message contained in it to the file in_msg_signed.bin stored. For decryption, the certificate of the recipient (receiver.pem) together with private key (receiver.key) needed
openssl ^ cms ^ -decrypt ^ -inform DER ^ -in in_msg_encrypted.bin ^ -out in_msg_signed.bin ^ -outform DER ^ -recip empfaenger.pem ^ -inkey empfaenger.key
To improve the readability of the command, line breaks of the Windows command line have been entered.
The arguments used have the following meaning:
Argument | Description |
---|---|
cms | OpenSSL shall process a CMS data structure (PKCS#7). |
-decrypt | This is an EnvelopedData data structure that is to be decoded. |
-inform THE | The CMS data structure is to be read in DER-coded (binary). |
-in msg_encrypted.bin | The file name of the message to be processed. |
-out in_msg_signed.bin | The file name of the message to be output. |
-outform THE | The decrypted message is to be output DER-encoded (binary). |
-recip recipient.pem | The CMS data structure is to be decrypted with the identity contained in the specified certificate. |
-inkey empfaenger.key | Reference to the private key of the recipient certificate. |
Check signature and output plain text
The following command displays the signature of the files stored in the in_msg_signed.bin contained CMS message and writes the plain text message to the file in_msg_plain.txt Saved.
openssl ^ cms ^ -verify ^ -inform DER ^ -CAfile CAChain.pem ^ -in in_msg_signed.bin ^ -out in_msg_plain.txt
To improve the readability of the command, line breaks of the Windows command line have been entered.
The arguments used have the following meaning:
Argument | Description |
---|---|
cms | OpenSSL shall process a CMS data structure (PKCS#7). |
-verify | This is a SignedData data structure that is to be verified. |
-inform THE | The CMS data structure is to be read in DER-coded (binary). |
-CAfile CAChain.pem | Contains the certificate chain of the used certificates to be able to establish the trust status to them. |
-in in_msg_signed.bin | The file in which the CMS message to be checked is stored. |
-out in_msg_plain.txt | The file to which the plaintext message should be saved. |
Data transmission
Transfer of data to the web service and authentication via certificate.
Here, too, two certificates (sender.pem and recipient.pem) are named in the examples for reasons of comprehension; in real operation, of course, it is the same certificate in both cases.
cURL is in current Windows 10 builds fixed part of the operating system and does not need to be installed separately.
Data retrieval
The signed and encrypted user data is sent as an MTOM object with the SOAP message.
curl ^ -k https://itsg.eservicet-drv.de/SPoC/ExtraService ^ --cert empfaenger.pem ^ --key empfaenger.key ^ --cacert CAChain.pem ^ --tlsv1.2 ^ -d @message.xml ^ -H "Content-Type: application/soap+xml" ^ -o Outfile.txt
To improve the readability of the command, line breaks of the Windows command line have been entered.
The arguments used have the following meaning:
Argument | Description |
---|---|
-k https://itsg.eservicet-drv.de/SPoC/ExtraService | The address of the web service. |
-cert recipient.pem | Authentication to the web service should be done with the specified certificate. |
-key receiver.key | Reference to the private key of the authentication certificate. |
-cacert CAChain.pem | Contains the certificate chain of the used certificates to be able to establish the trust status to them. |
-tlsv1.2 | Only Transport Layer Security (TLS) version 1.2 is to be used. |
-d @message.xml | The MTOM message containing the encrypted and signed user data. |
-H "Content-Type: application/soap+xml" | Specifies via HTTP header what kind of data is transferred. |
-o Outfile.txt | The output of the web service is redirected to the specified file. |
Sending data
The signed and encrypted user data is sent as an MTOM object with the SOAP message.
To improve the readability of the command, line breaks of the Windows command line have been entered.
curl ^ -k https://itsg.eservicet-drv.de/SPoC/ExtraService ^ -X POST ^ --cert sender.pem ^ --key sender.key ^ --cacert CAChain.pem ^ --tlsv1.2 ^ --data-binary @message.xml ^ -H "content-type: multipart/related; boundary=MIME-Multipart-Boundary;Content-ID=RootPart; charset=iso-8859-1;" ^ -o Outfile.txt
The arguments used have the following meaning:
Argument | Description |
---|---|
-k https://itsg.eservicet-drv.de/SPoC/ExtraService | The address of the web service. |
-X POST | POST is to be used as the HTTP method. |
-cert sender.pem | Authentication to the web service should be done with the specified certificate. |
-key sender.key | Reference to the private key of the authentication certificate. |
-cacert CAChain.pem | Contains the certificate chain of the used certificates to be able to establish the trust status to them. |
-tlsv1.2 | Only Transport Layer Security (TLS) version 1.2 is to be used. |
-data-binary @message.xml | The MTOM message containing the encrypted and signed user data. |
-H "content-type: multipart/related; boundary=MIME-Multipart-Boundary;Content-ID=RootPart; charset=iso-8859-1;" | Specifies via HTTP header what kind of data is transferred. |
-o Outfile.txt | The output of the web service is redirected to the specified file. |
Some troubleshooting tips
Message analysis
For the purpose of analysis and troubleshooting, it is very helpful to keep the certificate in the Windows certificate store along with the private key.
The message can then be evaluated with the following command:
certutil -dump {file}
The content of an encrypted CMS message can be directly extracted with the following command:
certutil -strip {file}
Related links:
External sources
- Security Interface (SECON) (Electronic data exchange in the statutory health insurance)
- Interface specification for XML-based, electronic data exchange with the pension insurance in the procedure Reha§301 (German Pension Insurance Association)
- Data Exchange in the German Health Service with CryptoSys PKI (D.I. Management Services Pty Limited)
- tinyHeb, An open source billing software for midwives
- RFC 5652 - Cryptographic Message Syntax (CMS) (Internet Engineering Task Force)